E-mail¶
DialoX bots can be configured to have conversations with users over e-mail as the primary messaging channel.
Channel configuration¶
For e-mail, SMTP is used as the main protocol. For sending emails, we need to provide an external SMTP server to send the email through, in order to prevent the email to be marked as spam.
There are two ways to configure an outbound email service: entering SMTP account
credentials directly, or using a Google account (sending and receiving email
through gmail). Both of these require configuring the integrations
file to add
a specific provider. Read more about OAuth integrations here.
Configuring SMTP credentials¶
Add the following to the integrations
yaml file:
- context: bot
provider: smtp
alias: smtp
description: "Outgoing email"
And publish the bot afterwards. You will see that an Integrations menu item has appeared in the menu, that will contain an "SMTP" item. When you click it, and click Configure, the following dialog box appears in which you need to configure the SMTP connection:
The SMTP server, username and password are usually things that your systems
administrator can provide to you. For Outlook.com / Microsoft accounts it is
usually smtp.office365.com
. The username is usually equal to the public email
address.
Configuring Gmail credentials¶
Add the following to the integrations
yaml file:
- provider: google
alias: google
description: Gmail SMTP
scope: https://mail.google.com/ email profile
context: bot
And publish the bot afterwards. You will see that an Integrations menu item has appeared in the menu, that will contain an "Gmail SMTP" item. When you click it, you can click Request authorization to start an OAuth flow which links a Google account to DialoX, giving it permission to send email through the gmail account. Google will warn you with the following screen:
It is good to know that our platform only uses these permissions to send email, we will not read existing emails from the gmail account nor will we ever delete any email messages from gmail.
Adding the email channel¶
Once an SMTP integration has been set up, it is now possible to add the email channel through the Channels page:
When the email channel is activated you are required to enter the following information:
- Sender name - the name of the sender as it will appear on the email, defaults to the bot title
- SMTP erver connection - the SMTP integration that you just set up in the step before this
- Conversation tracking - how the platform keeps track of conversations in email, which is either through a special email address or through a unique code in the email subject.
When the channels is added, a unique email address is assigned to your bot in
the form of identifier@bsqd.me
. Sending any messages to this address will
start or continue a conversation with the bot.
Creating a forwarding rule¶
In order to let the bot receive email messages at the public email address, you need to create a forwarding rule from the public email address to this email. This needs to be done at the SMTP provider (e.g. outlook or gmail).
Inbox integration¶
For email channels, the inbox is changed slightly so that operators can compose proper emails, including attachments, Cc and Bcc fields:
Runtime integration¶
In Bubblescript, emails are received as normal text messages, every time a user
sends an email, it is as if somebody sent a chat message. On the outgoing side,
every time the bot say
's a message, it is sent out as an email.
Receiving messages¶
When an email message is received for a bot, it is converted into a
%Bubble.Message
object which contains the email text and all of its metadata.
The .text
value of the message is the email body, stripped of HTML formatting
and stripped of any replies and e-mail signature.
The .data
value of the message contains e-mail metadata:
message_id
subject
date
to
- recipient listfrom
- recipient listcc
- recipient listattachments
- attachment listis_reply
- whether the incoming message was a reply or notfull_body
- the full, unstripped HTML or text body of the emailfull_body_mime
-text/plain
when the email was a plain text message, otherwisetext/html
.
Signature / replies are stripped on a best-effort basis; the text might still contain unwanted text.
Recipient list¶
The to
, from
and cc
fields of the message.data
contain list of
recipients. Each recipient has the following fields: email
, first_name
, last_name
.
Attachment list¶
Incoming attachments are stored in a list. Each of these attachments has the following fields:
url
- the URL where the attachment has been storedtype
- the type of attachment, one ofimage
,audio
,video
,file
.caption
- the original filename of the attachmentmetadata
- a map containing extra information, most notably the attachment MIME type (content_type
field), the filesize
and the originalfilename
.
Sending messages - basic¶
By using say
, you can send an email message to the user. Multiline strings are
converted into HTML paragraphs, and any
Markdown formatting is
converted into HTML:
dialog email_basic do
say "This sends a single email message"
say """
This sends another message. This time, we add
multiple paragraphs with text.
And this is the second paragraph.
You can also use **bold**, *italic*, et cetera formatting.
And include [links](https://www.example.com/) in your email.
And even an image:
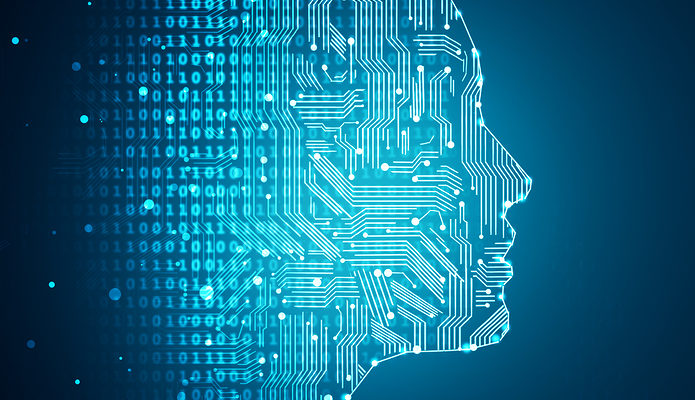
"""
end
Sending messages - advanced¶
To have more full control over the email, it is possible to use the special
"email"
template to send messages.
dialog email_template do
attachment = [
type: "image",
url: "https://www.botsquad.com/images/conversational_transformation.png",
caption: "ai.png"
]
show template("email",
body: "This is the markdown body text",
subject: "A email subject",
cc: "john@example.com, arjan@botsquad.com",
attachments: [attachment]
)
end
The email template supports the following arguments:
subject
- the subject to sendbody
- email body, markdown will be converted to HTMLattachments
- the attachment listto
- recipient list, or string with comma-separated email addressescc
- recipient list, or string with comma-separated email addressesbcc
- recipient list, or string with comma-separated email addressesraw_html_body
- raw HTML for the email body. When this is set, the normalbody
is not used and the custom HTML wrapper envelope is also not used.raw_text_body
will be used for the text version of the message if set; otherwise the HTML-version will be used, stripped of HTML tags.raw_text_body
- raw text message. When this is set, the normalbody
is not used. Whenraw_text_body
is not set, a plain text message will be sent out.extra_headers
- map with extra headers to be set on the email message
Custom HTML wrapper envelope¶
By default, outgoing email messages (except template messages with a
raw_html_body
) are wrapped with a standard HTML envelope. This envelope
contains the some basic markup of the email and styling.
This envelope can be overridden per bot by creating a HTML file called
email/html_template
.
The default HTML envelope looks like this:
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta name="x-apple-disable-message-reformatting" />
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
<meta name="color-scheme" content="light dark" />
<meta name="supported-color-schemes" content="light dark" />
</head>
<body>
{{{ body }}}
</body>
</html>
When writing a custom envelope, there are several template variables that can be used to fill the template in the appropriate places. These are the following:
body
— the original body of the message, e.g. the contents of the "say"last_user_message
— the last message the user said. This can be used to create an email message that looks like a reply.user
— All the properties of the user the email is sent to, e.g.user.first_name
,user.last_name
,user.email
, etc.conversation
— All the properties of the conversation. Amonst others,conversation.subject
is the current email subject; andconversation.operator
is the studio user that currently handes this message (can be used to template the closing of the message)
Mustache is the template syntax that is used to render the HTML template.
For instance, the body part of the HTML template could look something like this:
<body>
<p>Hello {{user.first_name}},</p>
<p>
{{{ body }}}
</p>
{{#conversation.operator}}
<p>Kind regards,</p>
<p>{{conversation.operator.first_name}}</p>
{{/conversation.operator}}
</body>
Deliver status tracking¶
The delivery of email messages can be tracked in realtime using REST API webhooks.
The following delivery status transitions are supported:
- For a message that gets sent and then is read by the recipient:
accepted
->delivered
->opened
- For a message that fails to deliver:
accepted
->failed
Note that when sending to multiple recipients, and one recipient fails, the
delivery status will be updated to failed
. It is not possible to see which
specific address had failed. In general, it is not advised to use the DialoX
platform as a mass-mailing tool.
REST API integration / example payloads¶
An example of an incoming e-mail, as it is received on the REST webhook:
{
"action": {
"id": "<10645acd-ac09-e03d-1e75-ec8ffd5cb86f@miraclethings.nl>",
"payload": {
"data": {
"attachments": [
{
"caption": "sunflower.jpg",
"from": null,
"metadata": {
"content_type": "image/jpeg",
"filename": "sunflower.jpg",
"size": 39078
},
"type": "image",
"url": "https://storage.googleapis.com/botsquad-assets/50e3c44b-262a-4e27-a48f-54c0e0a70f63/eb29aa37-db27-48cb-bcfa-c38e565067c3/E5MRXWQLG6RFXXRA725EPP65CG35F23F.jpg"
}
],
"cc": [],
"date": "Tue, 7 Dec 2021 14:14:48 +0100",
"from": [
{
"email": "arjan@miraclethings.nl",
"first_name": "Arjan",
"last_name": "Scherpenisse"
}
],
"full_body_mime": "text/plain",
"full_body": "Hello bot,\r\n\r\nThis is an email message that is received by the bot. It even contains \r\nan attachment.\r\n\r\ncheers, Arjan\r\n\r\n-- \r\nMiracleThings * / Interactive projects / creative solutions\r\nhttp://miraclethings.nl / arjan@miraclethings.nl / +31641322599\r\n",
"is_reply": false,
"message_id": "<10645acd-ac09-e03d-1e75-ec8ffd5cb86f@miraclethings.nl>",
"subject": "An example email message",
"to": [
{
"email": "iecrmy5we@msg.botsquad.com",
"first_name": null,
"last_name": null
}
]
},
"input_type": "email",
"text": "Hello bot,\n\nThis is an email message that is received by the bot. It even contains \nan attachment.\n\ncheers, Arjan",
"type": "text"
},
"time": "2021-12-07T13:14:06.273162Z",
"type": "user_message"
},
"bot_id": "eb29aa37-db27-48cb-bcfa-c38e565067c3",
"conversation_id": "qg72",
"user_id": "arjan@miraclethings.nl"
}
With the Operator Action REST Endpoint it is possible to send email messages to the user.
The payload for sending a basic text message from the bot to the user looks like this:
{
"action": {
"payload": {
"message": "Dear Arjan\n\nThank you for your mail, we will get back to you shortly.\n\nByebye!"
},
"type": "text"
}
}
And the payload for sending an email template message from the bot to the user looks like this:
{
"action": {
"payload": {
"attachments": [
{
"caption": "ai.png",
"type": "image",
"url": "https://www.botsquad.com/images/conversational_transformation.png"
}
],
"body": "This is the markdown body text",
"cc": "john@example.com, arjan@botsquad.com",
"subject": "A email subject",
"template_type": "email"
},
"type": "template"
}
}